Introducting: `go-credentials`
`go-credentials` is a library that can be used within Go to build and use simple credentials and configuration files for your CLI tools.
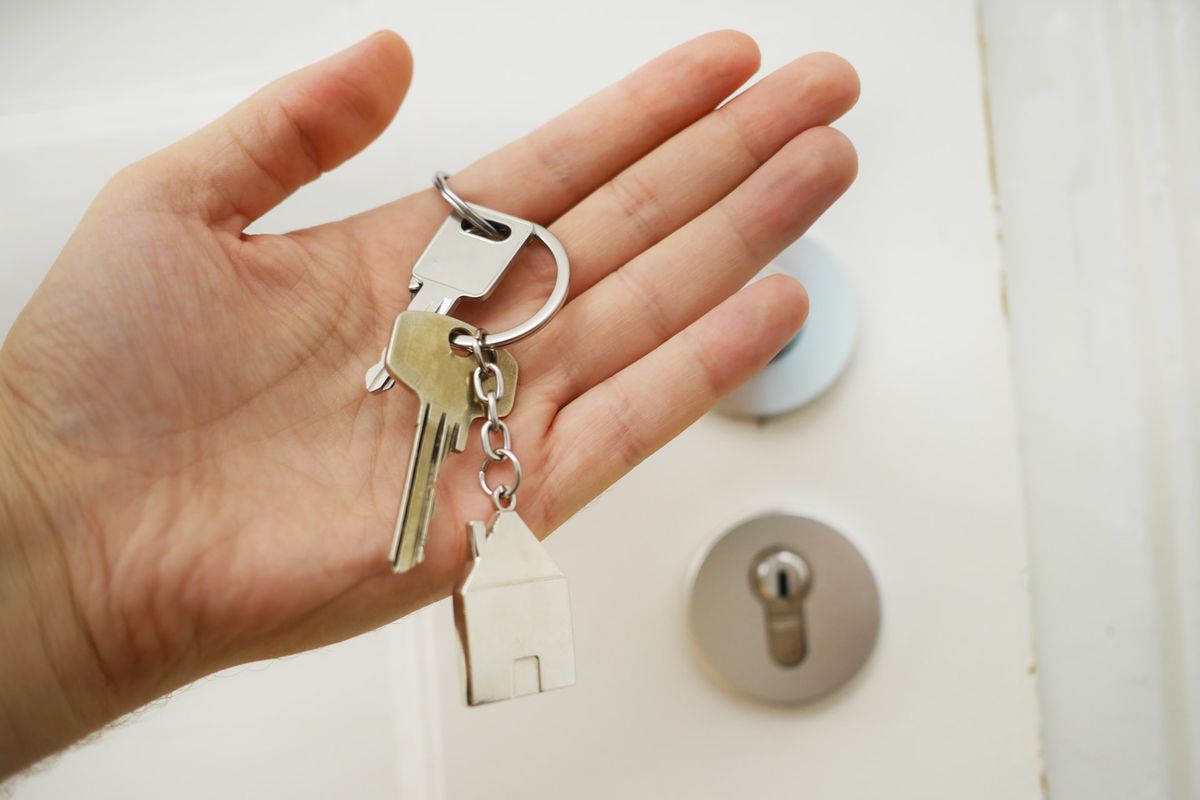
Today, I'm writing a simple post to talk about something I've worked on for the last few months. One of the things I really wanted was a way to manage credential files in Go, similar to how AWS manages credentials. But, I wanted it to have a bit more flexibility. Out of this was born go-credentials
.
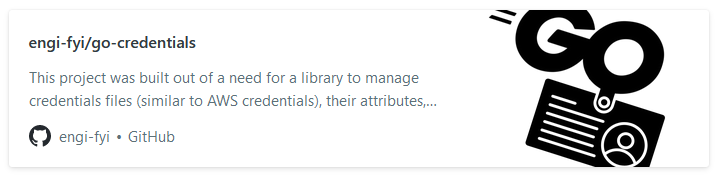
go-credentials
is a library for managing credentials using Go (who would have thought). There are three main concepts within go-credentials:
Factory
: this is how we generate our credential objects, and is where application settings are set.Credential
: what we're here for, our username and password.Profile
: authentication variables attached to a credential.
You can easily save and load credentials from files and the environment. At the moment, go-credentials
supports ini and json files, and environment variables. I'm planning on adding yaml soon in the future.
This has been a great project for me, as it really helped me to understand how Golang works properly and this is the first real open source work that I've done has felt like I've really produced something worthwhile.
If you'd like to get started, view the repository using the link above or you can also go to the GitHub Pages site. To get started, an example is below.
~/.gcea/credentials
[default]
username = test@engi.fyi
password = !my_test_pass==word
main.go
package main
import (
"github.com/engi-fyi/go-credentials/credential"
"github.com/engi-fyi/go-credentials/factory"
"fmt"
"time"
)
func main() {
myFact, _ := factory.New("gcea", false) // go-credentials-example-application
myFact.ModifyLogger("trace", true) // let's see under the hood and make it pretty.
myCredential, _ := credential.Load(myFact)
fmt.Printf("Username: %s\n", myCredential.Username)
fmt.Printf("Password: %s\n\n", myCredential.Password)
myCredential.Section("metadata").SetAttribute("last_updated", time.Now().Format("02/01/2006 15:04:05"))
myCredential.Save()
myCredential = nil
yourCredential, _ := credential.Load(myFact)
fmt.Printf("Username: %s\n", yourCredential.Username)
fmt.Printf("Password: %s\n", yourCredential.Password)
lastUpdated := yourCredential.Section("metadata").GetAttribute("last_updated")
fmt.Printf("Last Updated: %s\n", lastUpdated)
}
If you have any feedback, please feel free to leave an issue or a feedback request on GitHub. Or, if you like feel free to email os [at] engi.fyi.