Resize large images using ImageMagick and PowerShell
A simple tutorial on how to use PowerShell and the ImageMagick CLI to resize images.
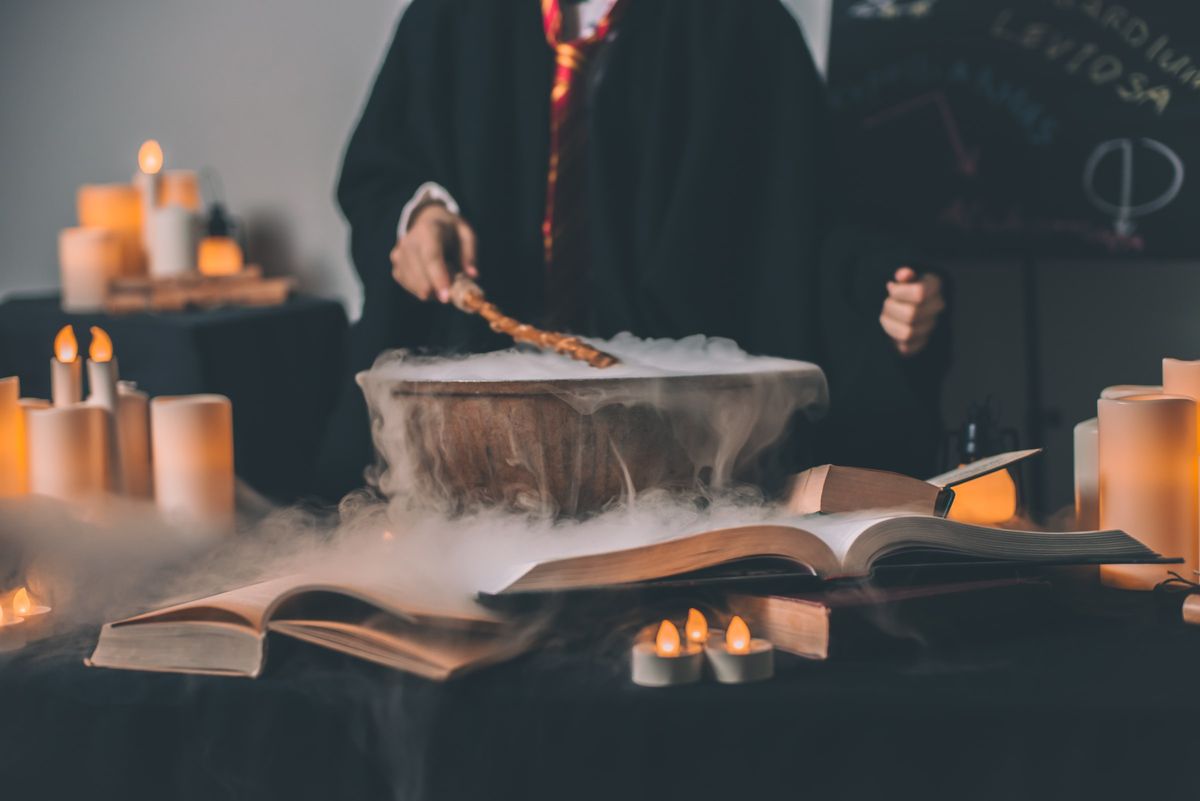
Sometimes you just write a script and need to save it for later; this is one of this times. This script simply lists all of the files in a directory recursively and if they are larger than certain breakpoints, will decrease the size of the image by a percentage amount. If it's run a few times, eventually the image will be around ~1800px high or wide. This script is quick and dirty, and does what it says on the tin - feel free to copy, modify, and enhance to your heart's content. Thanks to the team at ImageMagick for making some great tools.
Get-ChildItem -Recurse -File | Select -ExpandProperty FullName | ForEach-Object {
$FileName = $_
$Width = magick identify -format "%[w]" $_
$Height = magick identify -format "%[h]" $_
if ($Width -gt 6000 -or $Height -gt 6000) {
magick $FileName -resize 30% $FileName
} elseif ($Width -gt 5000 -or $Height -gt 5000) {
magick $FileName -resize 40% $FileName
} elseif ($Width -gt 4000 -or $Height -gt 4000) {
magick $FileName -resize 50% $FileName
} elseif ($Width -gt 3000 -or $Height -gt 3000) {
magick $FileName -resize 60% $FileName
} elseif ($Width -gt 2000 -or $Height -gt 2000) {
magick $FileName -resize 80% $FileName
} else {
"$FileName skipped"
}
}